In this learning phase we will discuss increment and decrement operations:
Increment operator ++
The unary increment operator ++ increments its operand by 1.
Here we have two types
1. Postfix increment operator x++
2. Prefix increment ++x
int a = 6;
int b = 4;
int c = a++; //value of c will be 6
int d=++a;
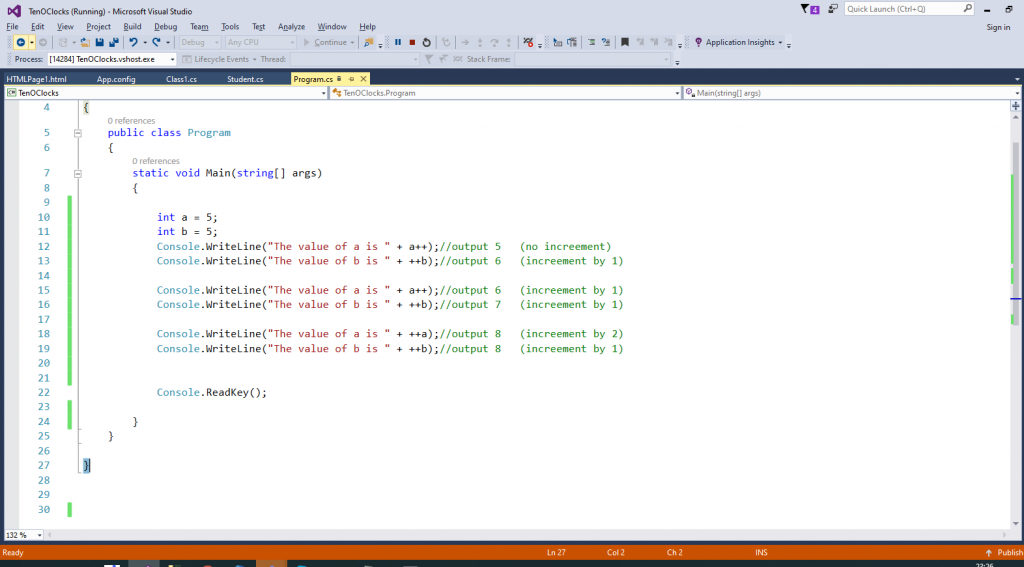
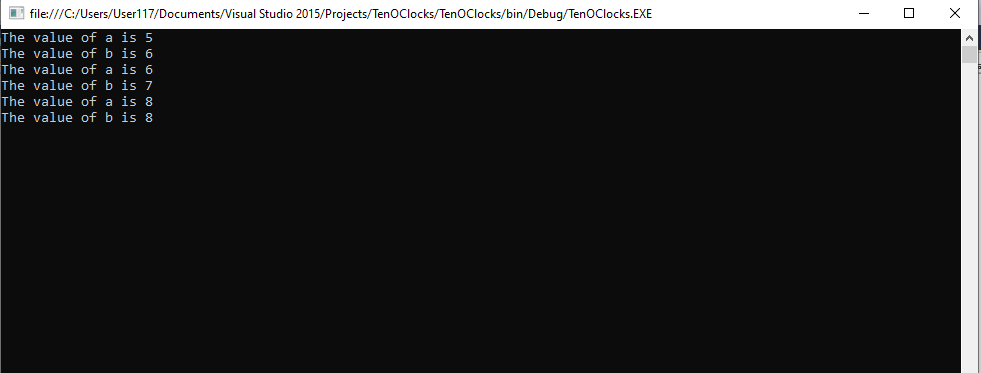
static void Main(string[] args)
{
int a = 5;
int b = 5;
Console.WriteLine("The value of a is " + a++);//output 5 (no increement)
Console.WriteLine("The value of b is " + ++b);//output 6 (increement by 1)
Console.WriteLine("The value of a is " + a++);//output 6 (increement by 1)
Console.WriteLine("The value of b is " + ++b);//output 7 (increement by 1)
Console.WriteLine("The value of a is " + ++a);//output 8 (increement by 2)
Console.WriteLine("The value of a is " + ++b);//output 8 (increement by 1)
Console.ReadKey();
}
output
The value of a is 5
The value of b is 6
The value of a is 6
The value of b is 7
The value of a is 8
The value of b is 8
**-when we increement the value of variable a by a++ it increements the value the next value of a
** -when we increement the value of variable a by ++a it directly increements the value of a
Decrement operator —
The unary increment operator — decrement its operand by 1.
1. Postfix decrement operator x–
2. Prefix decrement — x
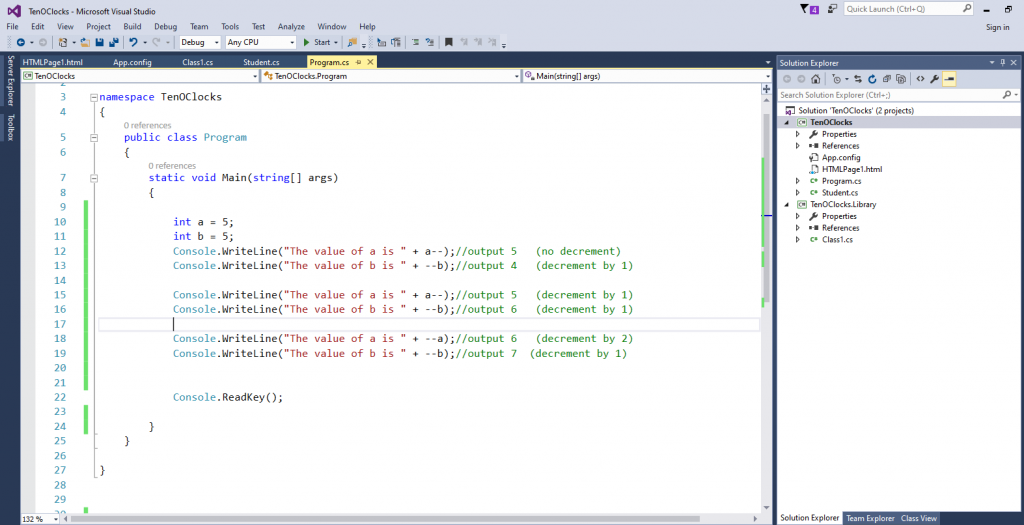
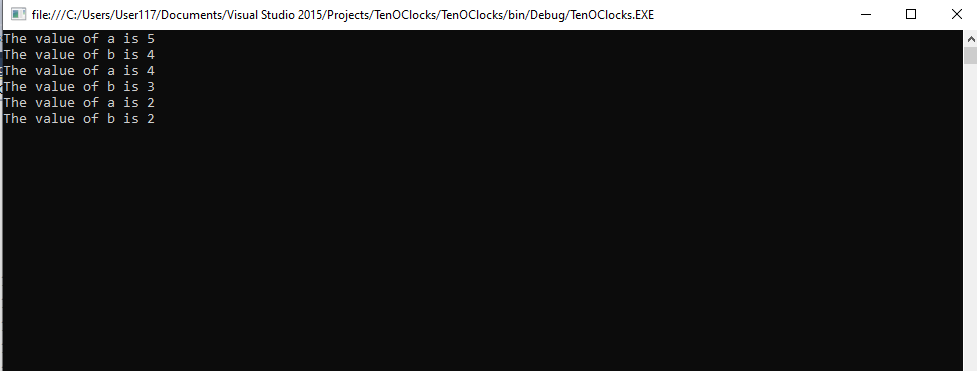
static void Main(string[] args)
{
int a = 5;
int b = 5;
Console.WriteLine("The value of a is " + a--);//output 5 (no decrement)
Console.WriteLine("The value of b is " + --b);//output 4 (decrement by 1)
Console.WriteLine("The value of a is " + a--);//output 4 (decrement by 1)
Console.WriteLine("The value of b is " + --b);//output 3 (decrement by 1)
Console.WriteLine("The value of a is " + --a);//output 2 (decrement by 2)
Console.WriteLine("The value of b is " + --b);//output 2 (decrement by 1)
Console.ReadKey();
}
output
The value of a is 5
The value of b is 4
The value of a is 4
The value of b is 3
The value of a is 2
The value of b is 2
**-when we decrement the value of variable a by a– it decrements its value for the next value of a
** -when we decrement the value of variable a by –a it directly instantaneously decrements the value of a
Sample Questions
if,
int a = 6;
int b =10;
Console.WriteLine(a++ + ++b);
Console.WriteLine(a++ + ++b + ++b +a);
Console.WriteLine(a++ + –b + b++ +a + b++);
Console.WriteLine(a– + ++b + ++b +a);
Solve and check the value of these by yourself .
C#- Programming Guide | Starting Phase Learning(8)
1 Comment